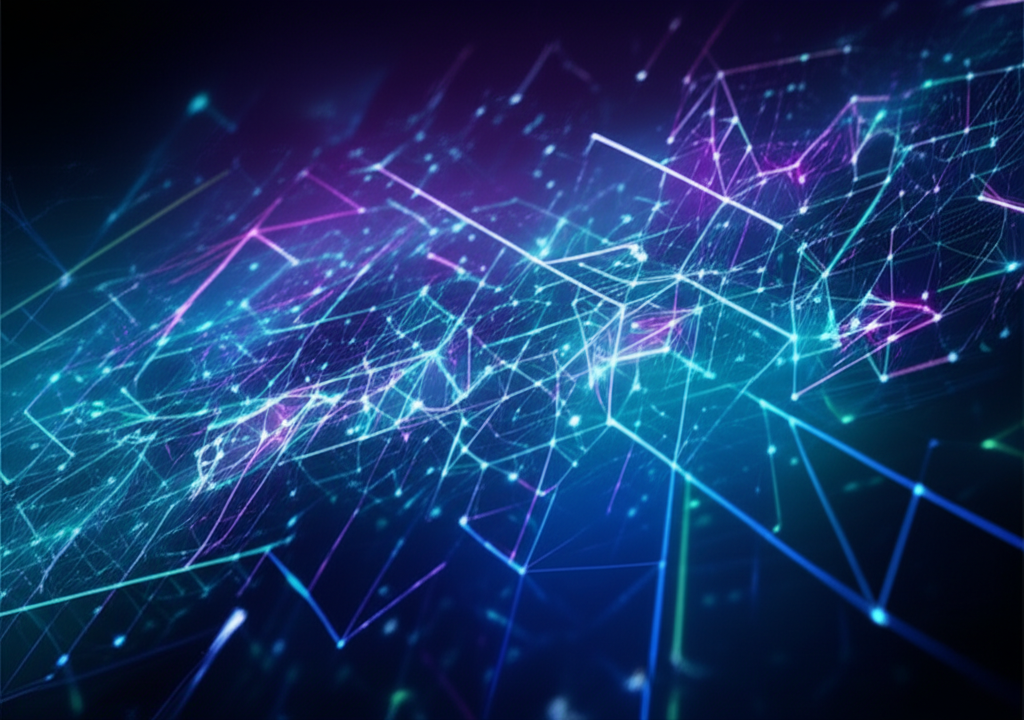
Getting Started with Next.js and TypeScript
Getting Started with Next.js and TypeScript
Next.js has emerged as one of the most popular React frameworks, providing a robust foundation for building modern web applications. When combined with TypeScript, it offers an even more powerful developer experience with static type checking, better tooling, and improved code quality.
Why Next.js?
Next.js solves many common challenges in React development:
- Server-side rendering (SSR) and static site generation (SSG) out of the box
- Automatic code splitting for faster page loads
- Built-in API routes to build your API alongside your front end
- Optimized image handling with the Image component
- Simplified routing with file-system based routing
Adding TypeScript to the Mix
TypeScript adds a robust type system to JavaScript, helping you catch errors early in development rather than at runtime. Benefits include:
- Early error detection
- Better IDE support with intelligent code completion
- Easier refactoring
- Self-documenting code
- Enhanced team collaboration
Setting Up a Next.js Project with TypeScript
Getting started is straightforward:
npx create-next-app@latest my-app --typescript
cd my-app
npm run dev
This creates a new Next.js project with TypeScript configuration already set up.
Key TypeScript Features for Next.js Development
Type-Safe Pages
import { GetStaticProps, NextPage } from 'next';
interface HomeProps {
posts: Post[];
}
const Home: NextPage<HomeProps> = ({ posts }) => {
return (
<div>
{posts.map(post => (
<PostCard key={post.id} post={post} />
))}
</div>
);
};
export const getStaticProps: GetStaticProps = async () => {
const posts = await fetchPosts();
return {
props: {
posts,
},
};
};
export default Home;
API Route Type Safety
import { NextApiRequest, NextApiResponse } from 'next';
export default function handler(req: NextApiRequest, res: NextApiResponse) {
if (req.method === 'POST') {
// Handle POST request
res.status(200).json({ message: 'Success' });
} else {
// Handle other methods
res.setHeader('Allow', ['POST']);
res.status(405).end(`Method ${req.method} Not Allowed`);
}
}
Best Practices
- Define clear interfaces for props, state, and API responses
- Use Next.js specific types like
GetStaticProps
,GetServerSideProps
, etc. - Create domain-specific type definitions in separate files
- Leverage TypeScript's utility types like
Partial
,Required
,Pick
, etc. - Configure tsconfig.json for strict type checking
Conclusion
The combination of Next.js and TypeScript provides a powerful foundation for building modern web applications. With type safety, improved developer experience, and the robust features of Next.js, you can build high-quality applications more efficiently than ever before.
If you're starting a new project or looking to improve an existing one, consider adopting this powerful technology stack.
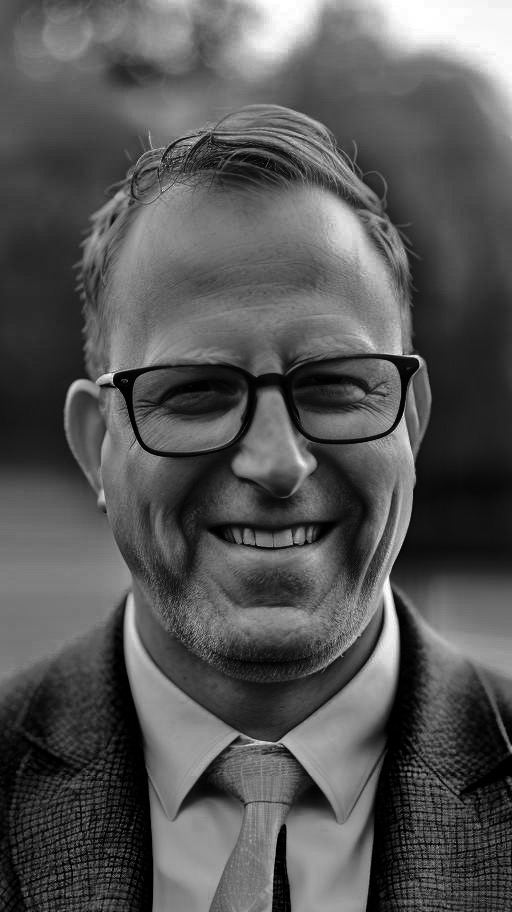
Jeff Rogers
Jeff is the founder and CEO of ATRIUMN, with over 20 years of experience building high-performance engineering teams and scaling startups.